Creating a Dark and Light Mode Toggle Button with JavaScript and CSS
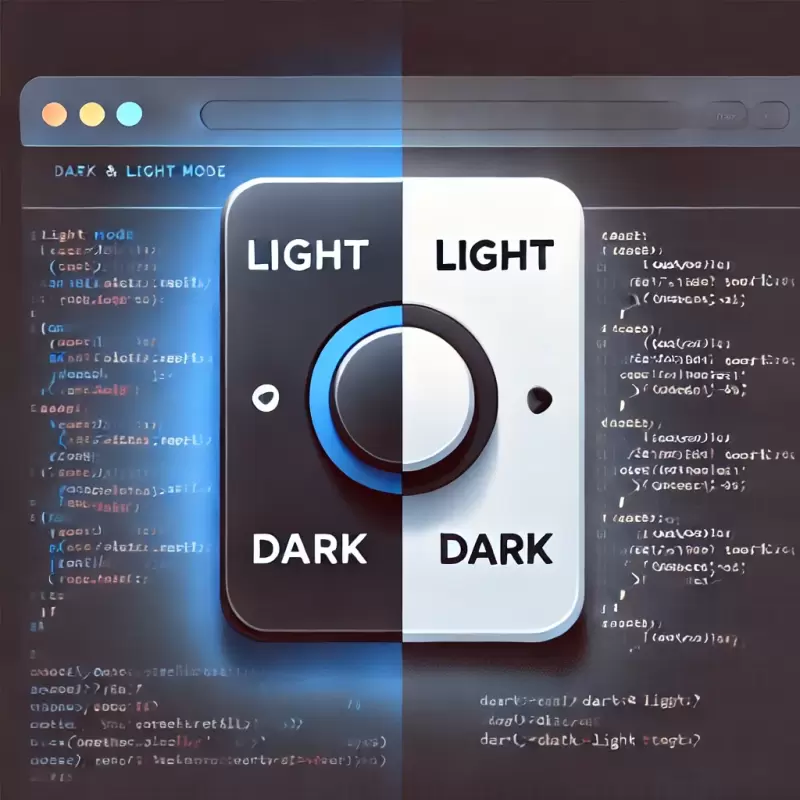
Nowadays, supporting Dark Mode has become a standard for modern websites, allowing users to select a color mode that best suits their viewing preferences. We can easily add a toggle button for users to switch between Light Mode and Dark Mode using JavaScript and CSS.
Step 1. Write the Basic HTML Structure
We start by creating the HTML structure for the webpage, including the button used for toggling the color mode. The HTML structure will include content and a button to switch color modes.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Toggle Dark Mode Example</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<h1>Hello, World!</h1>
<p>This page allows users to toggle between light and dark modes.</p>
<!-- Toggle button for switching modes -->
<button id="toggle-theme">Toggle Dark Mode</button>
<script src="script.js"></script>
</body>
</html>
Step 2. Write CSS for Light Mode and Dark Mode
Next, we’ll create the styles.css
file to define the styles for Light Mode and Dark Mode using the .light-mode
and .dark-mode
classes, which we’ll control with JavaScript.
/* Base styles */
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
transition: background-color 0.3s, color 0.3s;
text-align: center;
padding: 20px;
}
h1 {
font-size: 2em;
margin: 0.5em 0;
}
p {
font-size: 1.2em;
margin: 0.5em 0;
}
/* Styles for Light Mode */
.light-mode {
background-color: #ffffff;
color: #000000;
}
.light-mode button {
background-color: #000000;
color: #ffffff;
border: none;
padding: 10px 20px;
cursor: pointer;
border-radius: 5px;
}
/* Styles for Dark Mode */
.dark-mode {
background-color: #121212;
color: #ffffff;
}
.dark-mode button {
background-color: #ffffff;
color: #000000;
border: none;
padding: 10px 20px;
cursor: pointer;
border-radius: 5px;
}
/* Button hover effect */
button:hover {
opacity: 0.8;
}
Step 3. Write JavaScript for Toggling the Mode
Create the script.js
file to write JavaScript that checks the system color mode and manages mode toggling based on the user's selection.
// Check if the user has previously selected a color mode
const theme = localStorage.getItem('theme');
if (theme) {
document.documentElement.classList.add(theme);
} else {
// Set the default mode according to the system preference
if (window.matchMedia && window.matchMedia('(prefers-color-scheme: dark)').matches) {
document.documentElement.classList.add('dark-mode');
localStorage.setItem('theme', 'dark-mode');
}
}
// Function to toggle color mode
const toggleTheme = () => {
if (document.documentElement.classList.contains('dark-mode')) {
document.documentElement.classList.remove('dark-mode');
document.documentElement.classList.add('light-mode');
localStorage.setItem('theme', 'light-mode'); // Save the selected mode
} else {
document.documentElement.classList.remove('light-mode');
document.documentElement.classList.add('dark-mode');
localStorage.setItem('theme', 'dark-mode'); // Save the selected mode
}
};
// Attach the toggle function to the button
document.getElementById('toggle-theme').addEventListener('click', toggleTheme);
Code Explanation
-
Checking the System’s Color Mode
- When the webpage loads, JavaScript checks
localStorage
to see if the user previously selected a color mode. If so, the mode will be applied based on that saved setting. - If there is no saved setting, JavaScript will check the user’s system settings (if set to Dark Mode or Light Mode) and apply the corresponding mode to the webpage.
- When the webpage loads, JavaScript checks
-
The
toggleTheme
Function- This function checks if the current mode is
dark-mode
. If so, it switches tolight-mode
and saves this preference inlocalStorage
. - Conversely, if it’s in
light-mode
, it switches todark-mode
and saves the preference inlocalStorage
.
- This function checks if the current mode is
-
Connecting the Function to the Button
- When the user clicks the toggle button (
#toggle-theme
), thetoggleTheme
function is called, and the color mode changes immediately.
- When the user clicks the toggle button (
Summary
This article introduces how to create a toggle button for Dark Mode and Light Mode using JavaScript and CSS. It enables the webpage to check the system’s color mode, lets users manually switch modes, and saves the selected mode in localStorage
so the choice is remembered the next time they visit. This approach enhances the user experience by allowing them to control the appearance of the webpage according to their preferences.