Using async/await and setTimeout in JavaScript
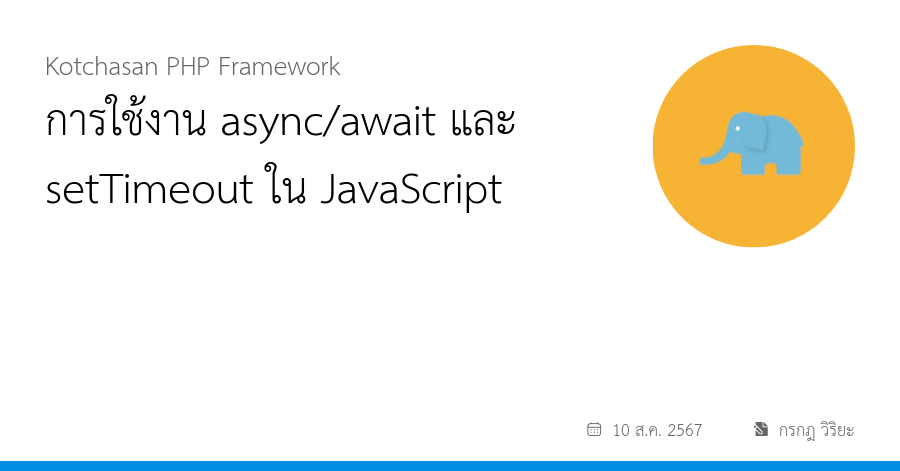
async/await
and setTimeout
are two widely used tools for handling asynchronous operations. While they serve different purposes and are applied in various contexts, there are certain aspects where they can be compared. In this article, we'll explore the similarities and differences between async/await
and setTimeout
and discuss when each method is most appropriate.async/await
async/await
is a feature in JavaScript that allows you to manage asynchronous functions that involve waiting for responses from other tasks. The async
keyword declares that a function is asynchronous, and the await
keyword pauses the function's execution until the awaited task is complete.Usage
async function fetchData() {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
}
When to Use
- When you need your code to execute in a specific sequence and wait for other tasks to complete before proceeding.
- For handling data processing that takes time, such as fetching data from an API, reading files, or performing long calculations.
setTimeout
setTimeout
is a function that schedules code to run after a specified delay. It does not block the execution of other code but will call the function after the delay.Usage
function delayedMessage() {
console.log('This message is delayed by 2 seconds');
}
setTimeout(delayedMessage, 2000); // Displays the message after 2 seconds
When to Use
- When you need to perform an action after a specified delay.
- To delay the execution of code or set a wait time before proceeding.
- To wait for other processes to finish before executing the specified function, by slightly delaying the command execution.
Similaritie
- Both
async/await
andsetTimeout
can control the timing of code execution, such as waiting for something to happen before proceeding. - Both are used for managing asynchronous operations but serve different purposes.
Differences
async/await
is used to manage the sequence of operations that need to wait for other tasks to complete, whilesetTimeout
is used to manage delays before executing a specified code.async/await
is suitable for handling tasks that require coordination and completion, whereassetTimeout
is ideal for tasks that need a delayed execution.
async/await
and setTimeout
are valuable tools in different contexts, depending on the purpose and requirements of the task. If you need to manage tasks that involve waiting for responses or coordinating sequences of operations, async/await
is the appropriate tool. However, if you need to introduce a delay before something happens, setTimeout
is the better choice.Example Usage of setTimeout When the Content of an Element Changes and Affects Its Size
When the content within an element changes, such as updating text or adding new content, it may cause the element's size to change. If you attempt to read the size of the element immediately after the content changes, you might get the old size because the DOM update hasn't completed yet. In this case, you can usesetTimeout
to wait until the DOM changes are fully processed before reading the correct size.Example Usage
// Suppose we have an element with the id "content"
const contentElement = document.getElementById('content');
// Change the content within the element
contentElement.innerHTML = '<p>Updated content that might change the size of the element.</p>';
// Read the size of the element right after the content changes
const originalHeight = contentElement.offsetHeight;
console.log('Original Height:', originalHeight); // May show the old size that hasn't been updated yet
// Use setTimeout to wait for the DOM changes to complete
setTimeout(() => {
const updatedHeight = contentElement.offsetHeight;
console.log('Updated Height:', updatedHeight); // Shows the updated size after the DOM changes
}, 0); // Delay of 0 milliseconds to allow the DOM update to complete
- When you change the content inside an element and immediately try to read the size (like
offsetHeight
oroffsetWidth
), you might get an incorrect value because the DOM update hasn't finished yet. - Using
setTimeout
with a delay of 0 milliseconds allows the DOM to finish processing the changes before you read the new size, ensuring that you get the correct value.